概要
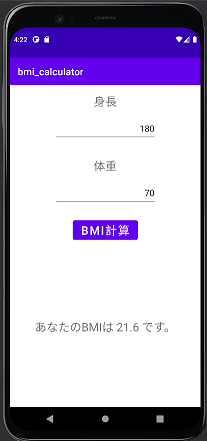
Androidアプリを作成する練習として、BMI計算機を作成しました。身長と体重を入力してBMI計算ボタンを押すとBMIが表示されます。
UIの作成
EmptyActivityテンプレートから作成を開始しました。
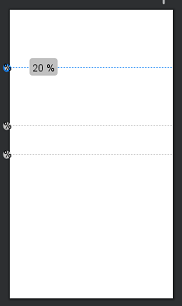
Guidelineを使用し画面を分割してから必要なビューを配置しました。
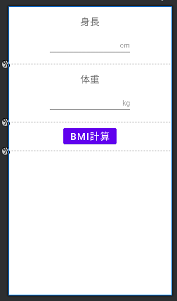
文字の表示はTextView、入力はEditText、ボタンはButtonをそれぞれ使っています。
findviewbyidでビューを取得する
findviewbyidメソッドでButtonを取得し、setOnClickListenerを使用してボタンが押された時の処理を登録します。
1 2 3 |
val calculationBtn = findViewById<Button>(R.id.calculation) calculationBtn.setOnClickListener { calculationBmi() } } |
BMIを計算する関数を作成する
calculationBmi関数は入力された身長と体重を取得してBMIを計算し、表示させる関数です。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
private fun calculationBmi() { // キーボードを非表示にする val view = currentFocus val inputMethodManager: InputMethodManager = getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager if (view != null) { inputMethodManager.hideSoftInputFromWindow(view.windowToken, 0) } val cm = findViewById<EditText>(R.id.inputHeight) val kg = findViewById<EditText>(R.id.inputWeight) // 身長と体重が入力されているかチェック when { cm.text.isEmpty() -> { cm.error = getString(R.string.heightError) } kg.text.isEmpty() -> { kg.error = getString(R.string.weightError) } else -> { val result = findViewById<TextView>(R.id.result) val bmi: Double = ((kg.text.toString().toDouble() / (cm.text.toString() .toDouble() / 100) / (cm.text.toString().toDouble() / 100)) * 100.0) .roundToInt() / 100.0 // formatメソッドで連結 result.text = getString(R.string.result).format(bmi) } } } |
BMI計算ボタンが押されると必要ではなくなったキーボードを非表示にしています。身長か体重が未入力の場合はEditTextのsetErrorメソッドを使用して入力をするようにエラー表示をさせています。
ソースコード
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
package com.bubudoufu.bmi_calculator import android.content.Context import android.os.Bundle import android.view.inputmethod.InputMethodManager import android.widget.Button import android.widget.EditText import android.widget.TextView import androidx.appcompat.app.AppCompatActivity import kotlin.math.roundToInt class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { setContentView(R.layout.activity_main) setContentView(R.layout.test) val calculationBtn = findViewById<Button>(R.id.calculation) calculationBtn.setOnClickListener { calculationBmi() } } private fun calculationBmi() { // キーボードを非表示にする val view = currentFocus val inputMethodManager: InputMethodManager = getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager if (view != null) { inputMethodManager.hideSoftInputFromWindow(view.windowToken, 0) } val cm = findViewById<EditText>(R.id.inputHeight) val kg = findViewById<EditText>(R.id.inputWeight) // 身長と体重が入力されているかチェック when { cm.text.isEmpty() -> { cm.error = getString(R.string.heightError) } kg.text.isEmpty() -> { kg.error = getString(R.string.weightError) } else -> { val result = findViewById<TextView>(R.id.result) val bmi: Double = ((kg.text.toString().toDouble() / (cm.text.toString() .toDouble() / 100) / (cm.text.toString().toDouble() / 100)) * 100.0) .roundToInt() / 100.0 // formatメソッドで連結 result.text = getString(R.string.result).format(bmi) } } } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 |
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/height" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/height" android:textSize="24sp" app:layout_constraintBottom_toTopOf="@+id/inputHeight" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <TextView android:id="@+id/weight" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/weight" android:textSize="24sp" app:layout_constraintBottom_toTopOf="@+id/inputWeight" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="@+id/guideline1" /> <EditText android:id="@+id/inputWeight" android:layout_width="wrap_content" android:layout_height="wrap_content" android:autofillHints="" android:ems="10" android:gravity="end" android:hint="@string/kg" android:inputType="number" android:minHeight="48dp" app:layout_constraintBottom_toTopOf="@+id/guideline2" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/weight" /> <EditText android:id="@+id/inputHeight" android:layout_width="wrap_content" android:layout_height="wrap_content" android:autofillHints="" android:ems="10" android:gravity="end" android:hint="@string/cm" android:inputType="number" android:minHeight="48dp" app:layout_constraintBottom_toTopOf="@+id/guideline1" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/height" /> <Button android:id="@+id/calculation" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/bmi" android:textSize="24sp" app:layout_constraintBottom_toTopOf="@+id/guideline3" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="@+id/guideline2" /> <TextView android:id="@+id/result" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="" android:textSize="24sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="@+id/guideline3" /> <androidx.constraintlayout.widget.Guideline android:id="@+id/guideline1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal" app:layout_constraintGuide_percent="0.2" /> <androidx.constraintlayout.widget.Guideline android:id="@+id/guideline2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal" app:layout_constraintGuide_percent="0.4" /> <androidx.constraintlayout.widget.Guideline android:id="@+id/guideline3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal" app:layout_constraintGuide_percent="0.5" /> </androidx.constraintlayout.widget.ConstraintLayout> |
1 2 3 4 5 6 7 8 9 10 11 |
<resources> <string name="app_name">bmi_calculator</string> <string name="height">身長</string> <string name="cm">cm</string> <string name="weight">体重</string> <string name="kg">kg</string> <string name="bmi">BMI計算</string> <string name="result">あなたのBMIは %s です。</string> <string name="heightError">身長を入力してください。</string> <string name="weightError">体重を入力してください。</string> </resources> |
最後までお読みいただきありがとうございました。